Guarding Routes: Securing Your Application with Laravel's Authentication System
Welcome to Topic 3 of Lesson 8: Laravel's Authentication System. In this topic, we unravel the art of safeguarding your application routes from unauthorized access. It's time to don your digital armor as we delve into the world of route authentication in Laravel.
The Importance of Route Guarding
Imagine a medieval castle that exposes all its entrances, allowing anyone to wander in freely. Chaos would ensue, jeopardizing the security and integrity of the kingdom. Similarly, in web development, protecting your application's routes is paramount. Laravel's authentication system empowers you to control access to different parts of your application, ensuring that only authorized users can pass through the castle gates of your web kingdom.
Utilizing Middleware
Laravel employs middleware as its primary tool for guarding routes. Middleware acts as a strong castle gatekeeper, scrutinizing each request before allowing passage. With Laravel's out-of-the-box middleware, you can easily authenticate users, enforce user roles and permissions, and perform various security checks.
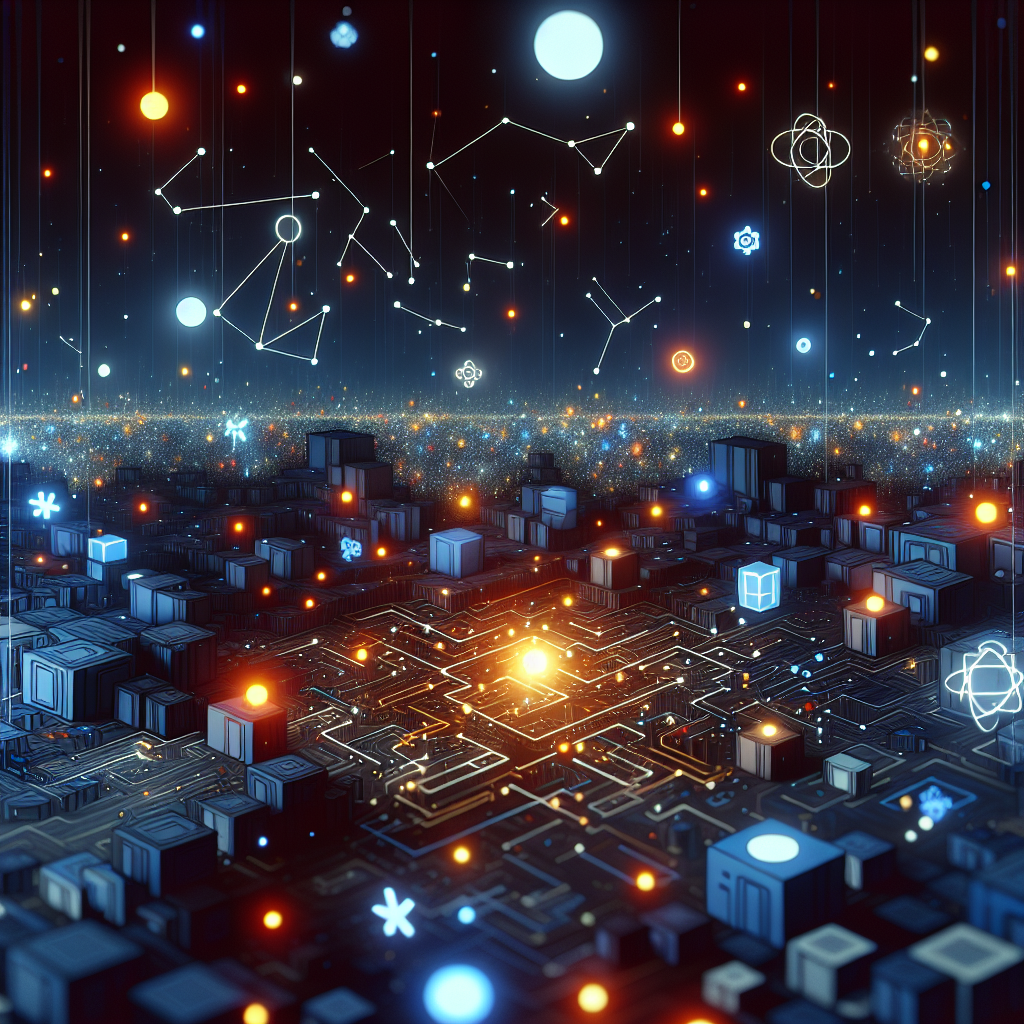
Applying Middleware to Routes
Applying middleware is as simple as wielding a master key. In your route definitions, you can indicate which middleware should safeguard the route:
Route::get('/secret', function () {
//
})->middleware('auth');
In the example above, the 'auth' middleware is employed to protect the '/secret' route. When a user attempts to access this route, Laravel's authentication system will kick in, verifying the user's credentials.
Creating Custom Middleware
If the built-in middleware doesn't meet your specific needs, Laravel gives you the freedom to forge your own. Custom middleware allows you to define custom security checks, logics, and actions, giving you complete control over route protection:
php artisan make:middleware CustomMiddleware
This artisan command creates a new custom middleware class. You can then define your desired security measures within the middleware's 'handle' method, customizing the authentication and authorization process.
Grouping and Applying Middleware to Route Groups
To streamline middleware application, Laravel lets you group routes and apply middleware to the entire group at once. This approach simplifies the task of guarding multiple related routes:
Route::middleware(['auth'])->group(function () {
Route::get('/dashboard', function () {
// Only authenticated users can access this route
});
Route::get('/profile', function () {
// Only authenticated users can access this route as well
});
});
In this example, both the '/dashboard' and '/profile' routes are protected by the 'auth' middleware. Laravel checks the user's authentication status before granting access to any of the routes within the group.
Conclusion: Fortifying Your Application's Defenses
You've reached the culmination of our exploration into route guarding with Laravel's authentication system. By understanding the underlying importance of securing routes and delving into middleware concepts, you've gained the power to control access to different parts of your Laravel application.
Apply these newfound skills wisely, ensuring the right routes are protected and only authorized users can tread upon your application's inner sanctum. Remember, with great power comes great responsibility. Continuously reassess your security measures, staying abreast of the latest best practices in web application security.
As you proceed further in your Laravel mastery, remember that vigilance is key to keeping your application's castle walls impenetrable. May your routes remain guarded and your users' data shielded on their thrilling quests through the web!