One-to-Many Relationships: The Backbone of Complex Data Interactions
Let's embark on an exciting quest into the realm of One-to-Many relationships in Laravel, where the intricate tapestry of data interconnectivity is woven. A One-to-Many relationship is a cornerstone of database architecture. It reflects the real-world scenarios where a single entity can be associated with multiple others. For instance, think of a mother duck with her line of ducklings or an author who scribes numerous books; this is the essence of One-to-Many.
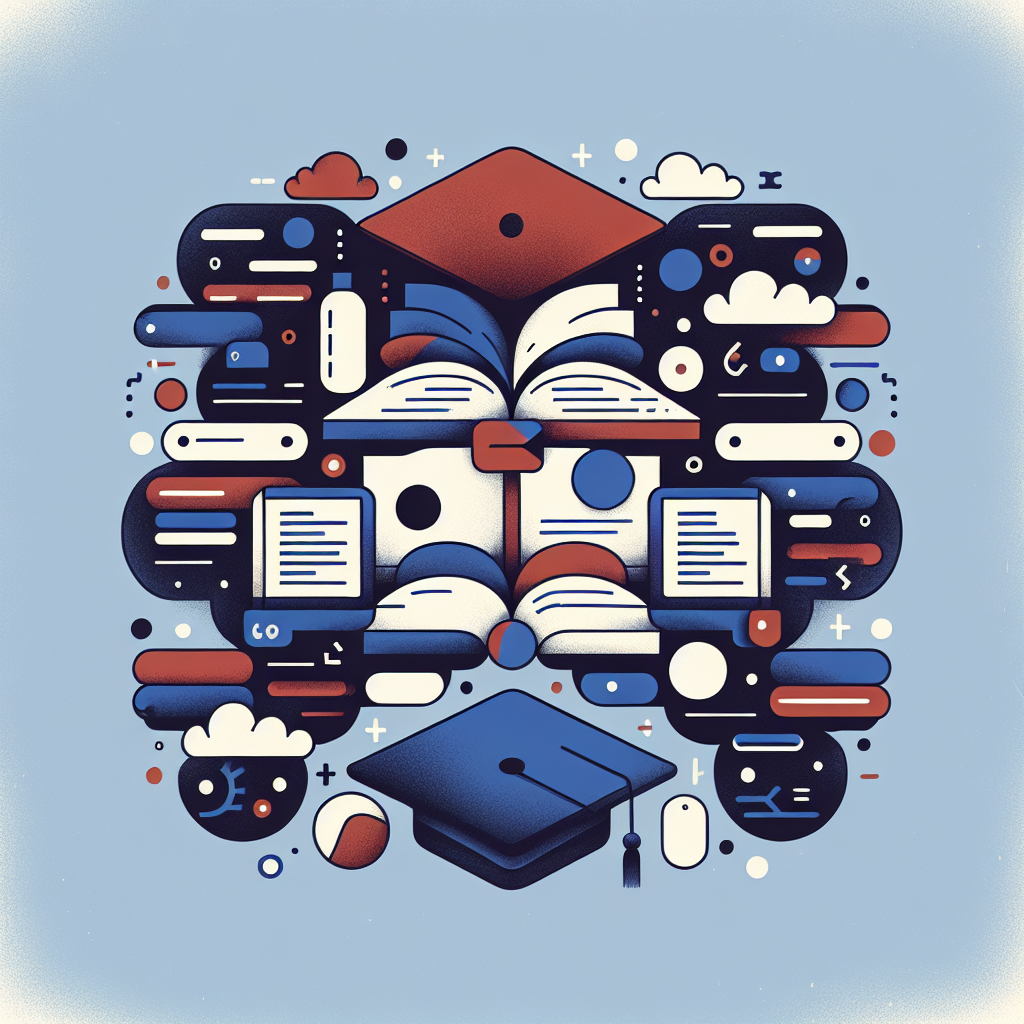
Deciphering One-to-Many Relationships
In the context of database relationships, understanding One-to-Many is pivotal. It allows one record in a table to be linked to multiple records in another. For you, the aspiring Laravel artisan, mastering this relationship means unlocking dynamic web application functionalities that truly reflect complex real-life data structures.
Defining One-to-Many in Eloquent
Within Laravel's Eloquent ORM, the beauty of defining relationships lies in its simplicity. A typical One-to-Many relationship requires you to define two complementary methods within related Eloquent models. The model on the 'One' side of the relationship will contain a method that returns the result of the 'hasMany' relationship method. Observe:
public function posts()
{
return $this->hasMany('App\Models\Post');
}
Here, a User model can be associated with many Post models. Eloquent gracefully manages the underlying complexity, intuitively setting up the proper database interactions.
Delving Deeper with Keys
Within Eloquent's 'hasMany' relationship, Laravel assumes the foreign key based on a conventional format. However, the real world can be unconventional, and Laravel prepares you for this by allowing you to specify a custom key if needed:
public function posts()
{
return $this->hasMany('App\Models\Post', 'custom_user_id');
}
This snippet explicitly defines which column to use as the foreign key, showcasing the framework's flexibility.
Conversing from the Many Side
Conversely, on the 'Many' side of the relationship, a method using the 'belongsTo' relationship method must be defined. This backlink completes the circle of association and allows the child model to recognize its parent:
public function user()
{
return $this->belongsTo('App\Models\User');
}
This inverse relation coins the connection, enabling you to retrieve the owner of each instance with effortless eloquence.
Harvesting Data with Eloquent's Elegance
The true power of these relationships wields when fetching related data. With Eloquent's eloquence, fetching a user's posts is as simple as:
$user = App\Models\User::find(1);
foreach ($user->posts as $post) {
echo $post->title;
}
In this portal of code, you effortlessly retrieve all posts related to a specific user, with Laravel doing the heavy lifting behind the scenes.
Crafting Real-World Scenarios
One-to-Many relationships are the bread and butter for representing real-world scenarios within your applications. They cater to commonplace examples, like a category having many products in an e-commerce site, a blog author owning many articles, or a teacher having many students. As a budding developer armed with Laravel, molding your applications to mirror these real-life contexts becomes a natural process.
Unleashing Advanced Techniques
Laravel also infuses your relationship arsenal with advanced features. Eager loading leverages the power of One-to-Many relationships efficiently, banishing the N+1 query problem into oblivion by loading relationships in advance. Furthermore, relationship methods can be chained with conditions to filter and shape the data you retrieve, bestowing even greater control over your model interactions.
Nurturing Best Practices and Performance
Along your journey through Laravel's One-to-Many relationships, embracing best practices ensures you craft the most optimal code. Naming conventions, proper indexing, and understanding when to use eager loading versus lazy loading all contribute to a well-performing application. Balancing these practices with Laravel's intuitive syntax allows you to manage complex data relationships with grace and performance.
A World of Possibilities with Laravel Relationships
As we conclude our excursion into One-to-Many relationships, remember, this is just one constellation in the galaxy of Laravel's capabilities. By mastering these relationships, you're setting the foundation for dynamic, data-driven applications that can evolve and scale with your users' needs. The journey to becoming a Laravel maestro continues, with each step forward in this course building upon the last, propelling you towards web development mastery.