The Vital Role of Routes in Laravel
Welcome to the core of web interaction in Laravel—routing. Imagine navigating the bustling streets of a city: every turn you take, every street you walk down, it's all part of a route that leads you to your destination. Laravel routes work similarly, guiding each request to the appropriate controller and function. Engaging with routing is engaging with the very heart of your application's functionality and flow.
Understanding the Basics:
Before we dive into coding, let's familiarize ourselves with what routes are. Routes in Laravel are the rules that tell your application what to do when a URL is accessed. They define how an application responds to various HTTP requests—such as GET for viewing a page, POST for submitting form data, and other HTTP verbs. Laravel's intuitive routing allows you to build both simple and complex routes for a dynamic user experience.
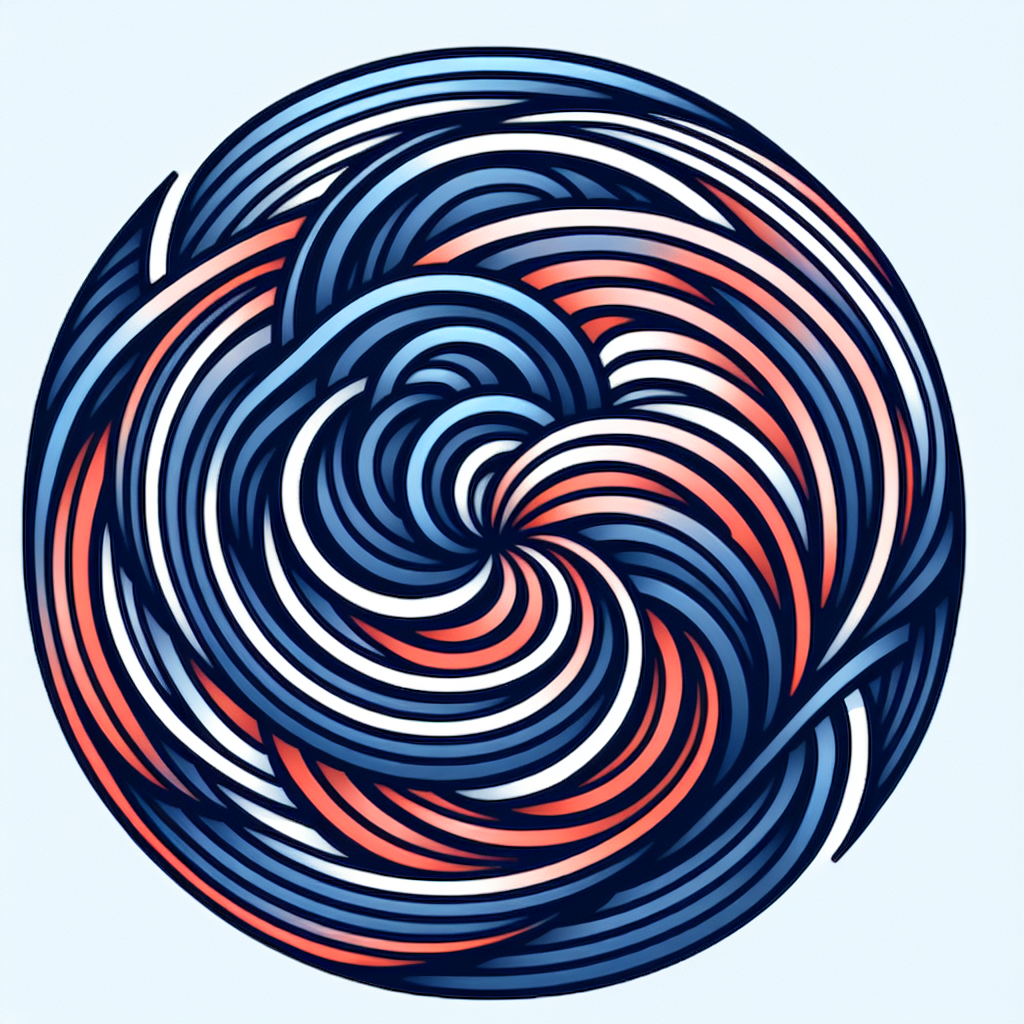
Defining Your First Laravel Route:
Now, let's draw our first map in Laravel. We start by defining routes within the 'web.php' file, located in the 'routes' folder. Think of this file as your city map where you plot out and label every journey that can be taken. A basic route might look something like this:
Route::get('/', function () {
return view('welcome');
});
This snippet is a simple guide directing traffic from the home ('/') URI to a view called 'welcome'. The route uses a closure to return the desired content, which, in this case, is a view.
Routes Lead to Actions:
Now, how does a route translate your instructions into action? Think of each route as an invitation to a specific part of your web application, calling out a response when interacted with. Whether you're asking to 'get' information displayed, 'post' data from a form, 'put' an update to a resource, or 'delete' something, routes are your go-to method for setting up these interactions.
One of the main principles to remember is that Laravel routes bridge the request to controllers. While simple closures are nifty for quick demonstrations, the true power comes with controllers that handle more extensive logic. By connecting a route to a controller, we write cleaner and more testable code. For example:
Route::get('/greet', 'GreetingController@index');
Exploring Route Patterns:
As we venture deeper into the world of routing, we realize that not all routes are static. Sometimes you need to capture information from the URL itself, like a user's name or an item's ID. These are often termed as 'dynamic routes' and are a fundamental feature you'll embrace as a developer.
The beauty of Laravel's routing is its flexibility. You can specify patterns and placeholders for parts of your routes that may change. For instance, if you want to capture a user ID:
Route::get('/user/{id}', function ($id) {
return 'User '.$id;
});
In the above route, the placeholder '{id}' dynamically accepts any user's ID and the corresponding function reacts accordingly, making your application interactive and user-specific.
Embrace Routing, Embrace Innovation:
Delving into Laravel's routing is the first step toward turning your digital ideas into reality. It represents more than just connecting dots; it's about crafting the journey that every user will take through your application. As you continue on this learning path, remember that each route you create is a new opportunity for innovation, a chance to shape how users experience and interact with your application.
Beginning Your Own Routing Adventure:
Are you ready to embark on this adventure and bring your digital landscapes to life with Laravel's routing? Let your creativity wander the paths you define, and watch as your web development potential flourishes through the art of routing.