Mastering Basic Routing in Laravel
Welcome to the exciting world of basic routing in Laravel! Just like building a network of roads to guide travelers to their destinations, basic routing in Laravel forms the foundation for navigating users through your web application. By understanding and comprehensively utilizing basic routing concepts, you'll be able to create dynamic, responsive, and user-centered web experiences.
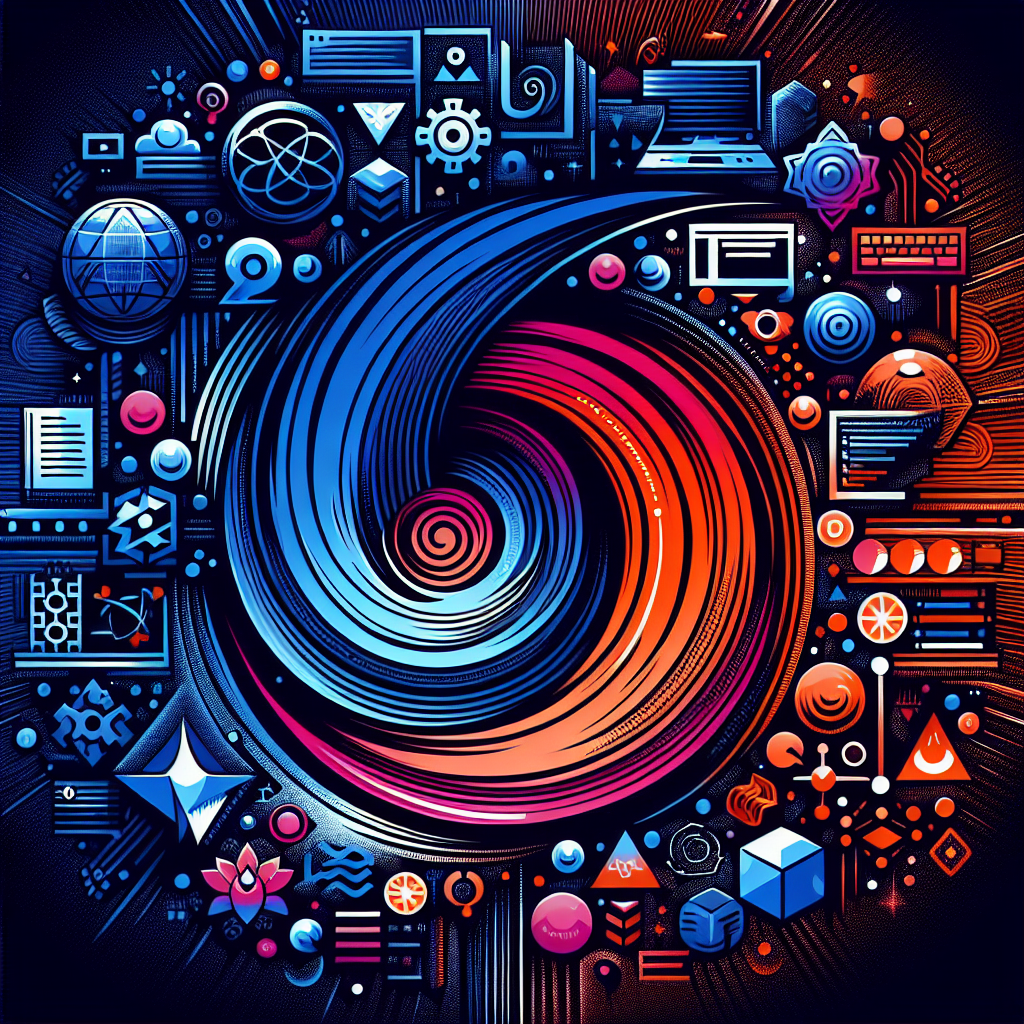
The Essence of Routing:
Routing is at the core of Laravel's architecture, providing a way to map URLs to specific actions in your application. It determines how your application responds to user requests, allowing you to display views, interact with databases, and perform various operations. With basic routing, you can create routes for different HTTP verbs (GET, POST, PUT, DELETE) and configure them to handle specific actions.
Defining Routes:
To start building routes in Laravel, you'll typically work within the web.php file located in the routes folder. Think of this file as the blueprint for your application's routing system. It's here that you define the different URLs (or routes) that users can access and specify the corresponding actions that should be taken.
A basic route in Laravel looks like this:
Route::get('/welcome', function () {
return view('welcome');
});
In this example, we're using the GET HTTP verb to define a route for the '/welcome' URL. When a user accesses this URL, Laravel will execute the specified function and return the 'welcome' view.
Working with Different HTTP Verbs:
As mentioned earlier, Laravel allows you to work with various HTTP verbs to handle different types of requests. The 'Route' facade provides methods for defining routes for these verbs, such as 'get', 'post', 'put', and 'delete'.
For instance, if you want to handle a POST request to submit a form, you can define a route like this:
Route::post('/submit', function () {
// Handle form submission logic here
});
By utilizing the appropriate HTTP verbs, you can create routes that respond dynamically to specific actions and behaviors within your application.
Passing Parameters:
Routes in Laravel can also include dynamic parameters, enabling you to create more flexible and personalized experiences for your users. Parameterized routes capture specific values from the URL and make them available to your application logic.
Here's an example of a route that captures a user's ID:
Route::get('/user/{id}', function ($id) {
// Access the user ID and perform operations
return view('user.profile', ['user' => $id]);
});
In this case, the route captures the value of 'id' from the URL and passes it as a parameter to the closure. You can then use this parameter within your function to fetch the relevant user data and display it in the 'user.profile' view.
Summary:
Basic routing is a powerful tool that allows you to define routes and handle user requests in your Laravel application. By understanding and applying basic routing concepts, you can effectively navigate users through your web application while providing them with dynamic and personalized experiences. Whether you're rendering views, processing form submissions, or accessing specific resources, basic routing equips you with the skills to create robust and user-friendly web applications.