Unlocking the Power of Route Parameters in Laravel
Imagine being able to create dynamic and personalized routes in your Laravel application, where different URLs lead to specific content or actions based on user input. This is made possible through the utilization of route parameters. With route parameters, you can capture and utilize dynamic values from the URL, opening up a world of possibilities for creating interactive and customizable web experiences.
Understanding Route Parameters:
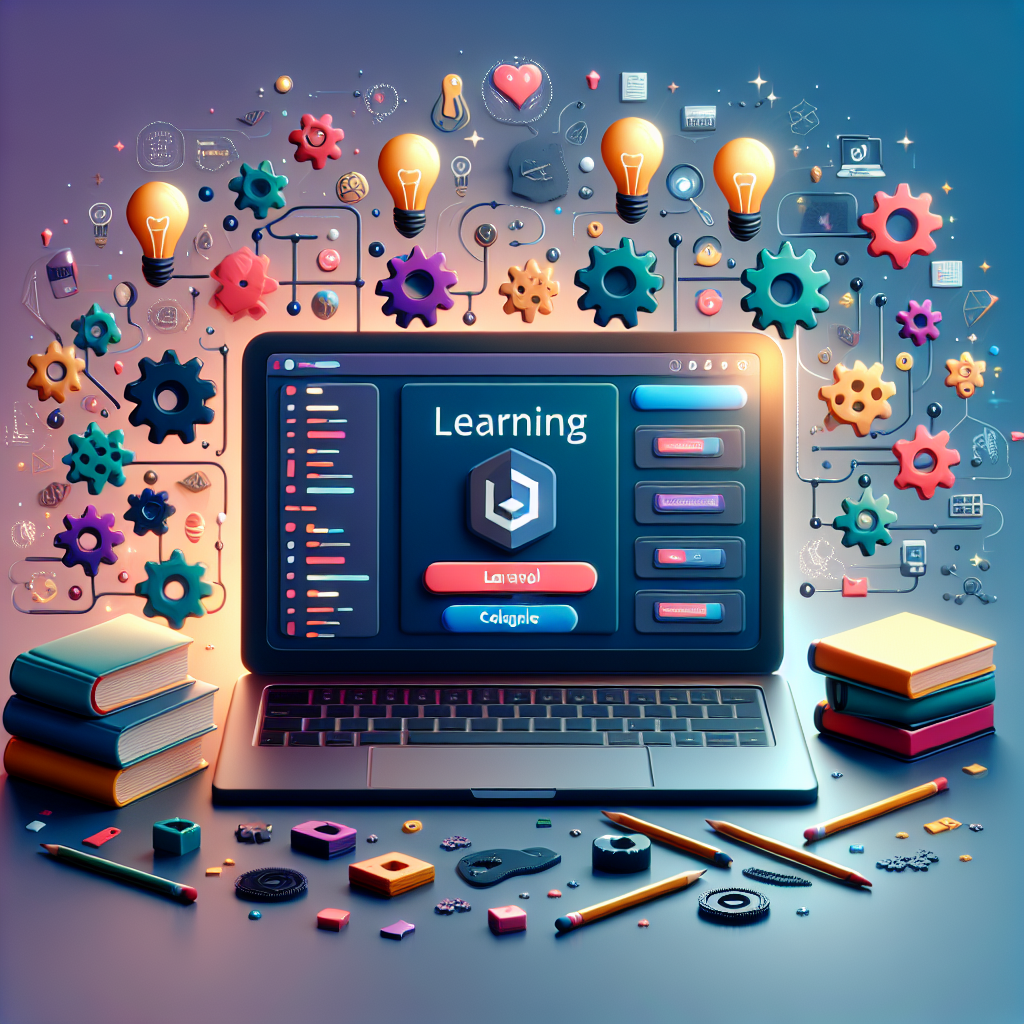
In Laravel, route parameters are placeholders within the route definition that capture specific parts of a URL and pass them as arguments to your application logic. By encapsulating dynamic segments of a URL, you can create routes that respond to various user inputs and provide dynamic content.
Defining Route Parameters:
To define a route parameter in Laravel, you enclose the placeholder within curly braces ('{}'). For example, consider the following route:
Route::get('/users/{id}', function ($id) {
// Retrieve user with specified ID
return view('user.profile', ['user' => $id]);
});
In this route, we have defined a parameter named 'id' within the '/users/{id}' URL. When a user visits a URL matching this pattern, Laravel captures the corresponding value and passes it as an argument to the closure. You can then utilize the parameter within your logic to retrieve relevant data, such as fetching a user with the specified ID and displaying their profile.
Passing Multiple Route Parameters:
Laravel allows you to capture multiple route parameters within a single route definition. Simply include additional placeholders within the URL and pass the corresponding arguments to your closure. For example:
Route::get('/users/{id}/posts/{postId}', function ($id, $postId) {
// Retrieve user and post with specified IDs
return view('post', ['user' => $id, 'post' => $postId]);
});
In this case, the route captures both the 'id' and 'postId' parameters, allowing you to retrieve and display data associated with both the user and the specific post.
Assigning Default Values:
Route parameters in Laravel can also have default values, which are useful when certain values are optional or when you want to provide a fallback. To assign a default value, you can specify it within the route definition itself. Here's an example:
Route::get('/users/{id?}', function ($id = null) {
// Retrieve user with specified ID (or default to null)
return view('user.profile', ['user' => $id]);
});
In this route, the 'id' parameter is marked as optional with the '?' symbol. If the parameter is not provided in the URL, Laravel will default it to null. This allows for greater flexibility in constructing routes that can handle a wide range of user scenarios.
Validating Route Parameters:
Laravel provides built-in validation mechanisms for route parameters, ensuring that the data passed through the URL meets your specified criteria. You can apply validation rules using Laravel's validation features, guaranteeing that the captured values are appropriate for your application's needs. Proper validation helps maintain the integrity and security of your routes and provides a streamlined user experience.
Integrating Parameters with Controllers:
While using closures to define routes is effective for simple purposes, leveraging controllers is a best practice for managing more complex application logic. By connecting routes to controllers, your code becomes more organized, modular, and testable.
In Laravel, you can easily link routes to controllers using the 'Route::' syntax. For example:
Route::get('/users/{id}', 'UserController@show');
This route maps to the 'show' method within the 'UserController', allowing you to centralize and encapsulate the logic for retrieving and displaying user data. Controllers provide a structured way to handle route parameters and perform the necessary operations based on the captured values.
Summary:
Route parameters enhance the versatility and interactivity of your Laravel application by allowing you to capture dynamic values from the URL. By utilizing route parameters, you can create personalized and adaptable routes that respond to user input. Whether you're building user profiles, displaying specific content, or filtering data, the power of route parameters offers endless possibilities for creating robust and engaging web applications.