Exploring the Efficiency of Named Routes in Laravel
Welcome to the topic of Named Routes in Laravel, where efficiency, readability, and flexibility in your web applications become the gold standard. In the marvelous journey of web development with Laravel, named routes are akin to having a personalized GPS system. They allow you to specify unique identifiers for routes, making it easier to generate URLs or redirects to specific parts of your application.
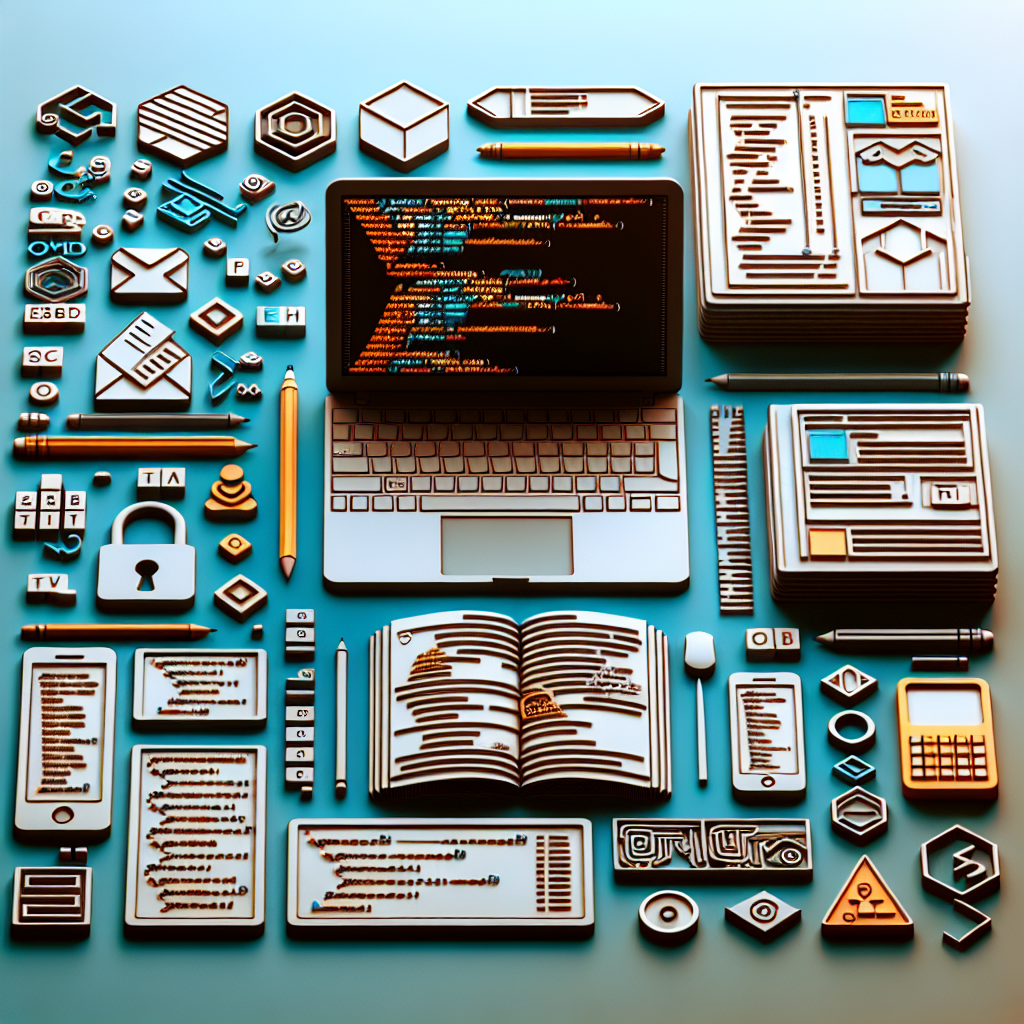
Benefits of Named Routes:
Using named routes comes with a myriad of benefits, notably the ease of maintaining your code. Imagine you need to update the URI of a route used across various views or actions. Without named routes, such a change could lead to a laborious search-and-replace task. However, with named routes, you update the URI in a single location, and voila—every reference stays intact and automatically adapits to your changes.
How to Define a Named Route:
Defining a named route in Laravel is a piece of cake. Assign a name to a route by chaining the 'name' method onto the route definition:
Route::get('/user/profile', function () {
// Your code here
})->name('profile');
Now, you have a route to the user's profile that can be referenced using the name 'profile'. This simplification in route referencing leads to cleaner, more expressive code when generating URLs or redirects.
Generating URLs to Named Routes:
The true power of named routes is harnessed when generating URLs. Instead of hardcoding URLs in your application, Laravel's 'route' function allows you to generate complete URLs based on the name of the route:
$url = route('profile');
This approach is not only neat and efficient, but it also protects your application from breaking changes if the route's URI is later altered.
Redirecting to Named Routes:
Alongside generating URLs, named routes are immensely useful for implementing redirects within your application. By using the 'redirect' function in conjunction with a route's name, your code gains clarity and maintainability:
return redirect()->route('profile');
This code sends the user to the 'profile' route without any need to know the underlying URI. It is as dynamic as it is reliable, reinforcing the robustness of your application.
Named Routes With Parameters:
Named routes are not limited to simple cases; they readily accept parameters as well. When you have dynamic URLs that require parameters, the 'route' function makes it a breeze to pass these values:
$url = route('user.show', ['id' => 1]);
This generates a URL to the 'user.show' route, including the provided user ID. The fluidity with which this operates saves you from concatenating strings and possibly making errors.
Grouping Named Routes:
As your application grows, organizing related routes becomes essential. Laravel provides route groups to keep your routes neat and manageable. Named routes can be grouped together, and any route names defined within the group are automatically prefixed:
Route::prefix('admin')->name('admin.')->group(function () {
Route::get('/users', function () {
// Code for admin users route
})->name('users');
});
In this group, the 'users' route can be referenced as 'admin.users', demonstrating the structured organization available to you using Laravel's routing capabilities.
Checking for Active Named Routes:
Laravel's 'request' function can check for active named routes, allowing you to apply conditional logic or styling within your application, such as highlighting an active menu item. This simple yet powerful functionality contributes to dynamic and user-friendly interfaces.
Embracing the Simplicity and Power of Named Routes:
As we wrap up our session on named routes, it's clear that this feature is a testament to Laravel's commitment to developer happiness and application maintainability. Named routes streamline and decouple your route references from their URIs, saving you time and future-proofing your applications. Understand and utilize named routes, and watch how they transform the way you write and maintain your code.