Elevating Your Routing Game with Route Groups
Imagine orchestration where every section of a grand symphony comes together in harmonious melody. This is akin to the concept of route groups in Laravel, a powerful feature that enables developers to organize numerous routes under a common theme or prefix, sharing attributes such as middleware, names, and namespace. As we dive into route groups, think of them as the framework for a structured, maintainable, and scalable routing system, harmonizing your Laravel application’s routing ensemble.
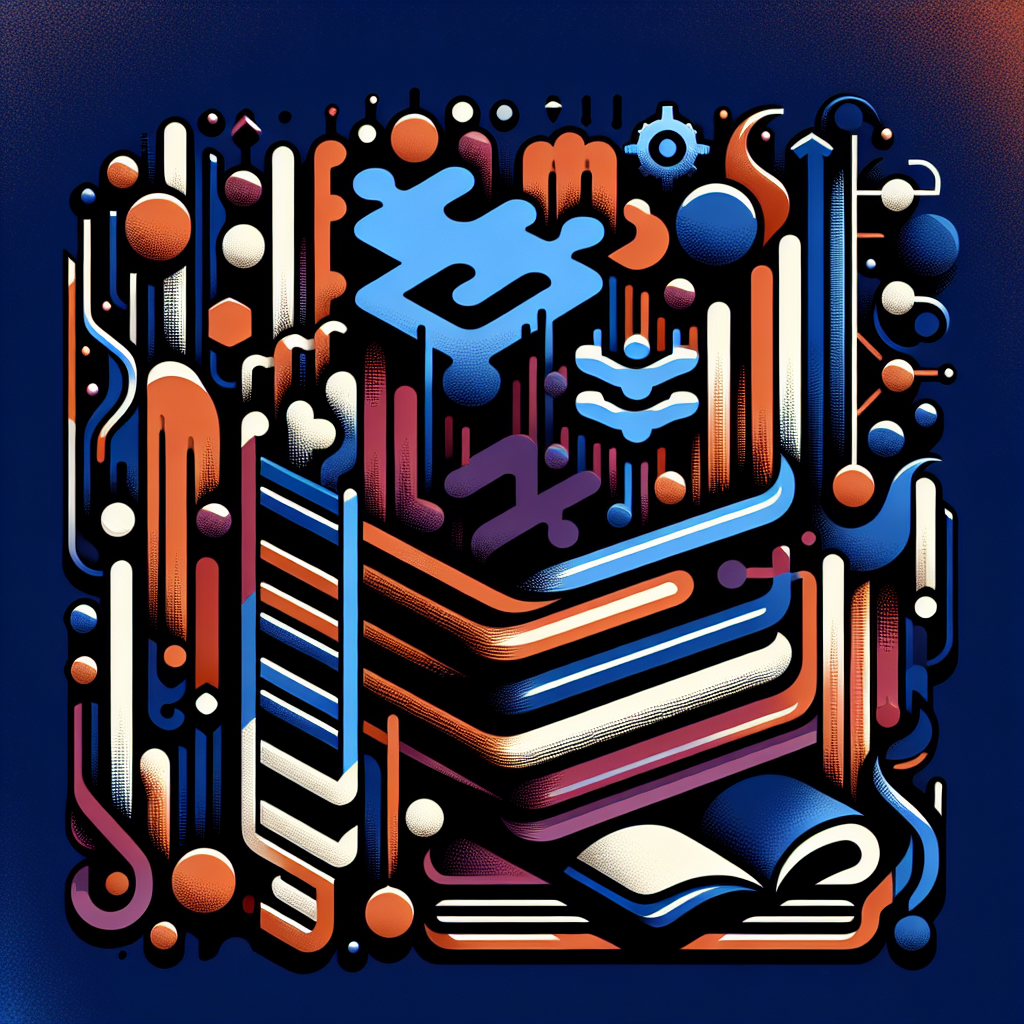
The Symphony of Grouped Routing:
Route groups empower you to manage a bulk of routes that fall into specific categories—such as admin routes, API routes, or routes that are protected by authentication. With route groups, you can apply attributes to multiple routes within a group, all in one swoop. This helps in writing less repetitive code, simplifying your routing files, and making your web development workflow more efficient.
Composing Route Groups:
To declare a route group in Laravel, you use the 'Route::group' method and pass an array of attributes and a closure containing the routes. You'll feel the magic unfold as routes that were once scattered come together to form a cohesive collection:
Route::group(['prefix' => 'admin', 'middleware' => 'auth'], function () {
Route::get('/', 'AdminController@index');
// More admin routes...
});
In this example, each route within the group inherits the 'admin' prefix and 'auth' middleware automatically, keeping your routing sleek and DRY (Don't Repeat Yourself).
Setting the Stage with Prefixes:
In a group, the 'prefix' attribute adds a common URI segment to the group's routes. Picture it as the entrance to a hall within a castle, where everything past that door is part of the same majestic chamber. It's particularly handy for creating a section of an application, like an admin dashboard, localized by a shared URL segment:
Route::group(['prefix' => 'dashboard'], function () {
// Dashboard related routes go here.
});
Now, all routes within this group will be automatically prefixed with '/dashboard', establishing a dedicated namespace for these routes in the global URL space.
Ensuring Security with Middleware:
Middleware in route groups serves as the guardians of your routeways, ensuring certain conditions, such as authentication or logging, are met before the group's routes are accessed. Applying middleware to a group is efficient, providing a simple way to protect a collection of routes:
Route::group(['middleware' => 'auth'], function () {
// Routes that require the user to be authenticated.
});
By attaching 'auth' middleware, every route within the group checks for an authenticated user session—no need to attach middleware to each route individually.
Harmonizing Routes with Namespaces:
When dealing with controllers in route groups, the 'namespace' attribute becomes the conductor, determining which PHP namespace the group's controllers will reside in. It provides a clear organizational structure, especially when dealing with a variety of controllers spread across your application:
Route::group(['namespace' => 'Admin'], function () {
// Routes that utilize controllers within the 'App\Http\Controllers\Admin' namespace.
});
This approach is akin to arranging your controllers into dedicated sections of an orchestra, allowing for easier management and a logical grouping of actions.
Organizing with Subdomain Routing:
Laravel's routing capabilities are not just limited to URI-based groups. You can also group your routes by subdomains, creating subspaces within your application's domain. This is perfect for applications that are divided into major sections accessible via different subdomains:
Route::group(['domain' => '{account}.myapp.com'], function () {
// All routes that are part of a given subdomain.
});
This group configuration directs all traffic for a specific subdomain to the set of routes, maintaining a logical separation in your application's routing logic.
Fluent Routing:
One of Laravel's deep-seated commitments is to provide a fluent, intuitive API for developers. Route groups are no exception. The ability to chain methods together to configure a route group offers a delightful, fluid interface that makes your routing declarations read like well-written prose,
Mastering Route Groups:
By mastering route groups, you empower your Laravel application with well-organized, maintainable routes, allowing you to manage your codebase more efficiently as it grows. This opens a realm of possibilities, enabling beginners and seasoned developers alike to build sophisticated applications with complex routing requirements. Embrace the harmony that Laravel's route groups bring to your projects, and watch as it transforms your routing composition into a masterpiece.