Unlocking Laravel's Superpower: Route Model Binding
Embark on a journey to discover Route Model Binding, the hidden gem of Laravel's routing capabilities. Route Model Binding simplifies how you interact with models when handling route parameters, saving you precious lines of code and potential for errors. This powerful feature elegantly bridges your routes to your database, making your code more expressive and efficient. Just like a master key that unlocks multiple doors, Route Model Binding enhances your Laravel toolkit by unlocking seamless integration between routes and models.
What is Route Model Binding?
Route Model Binding is, at its core, a miraculous way of injecting model instances directly into your routes. Whenever a route requires a specific model instance, Laravel graciously serves it up on a silver platter, based on the corresponding database column, usually the primary key. This feature eradicates the need for manually querying the database to retrieve the model in your route actions.
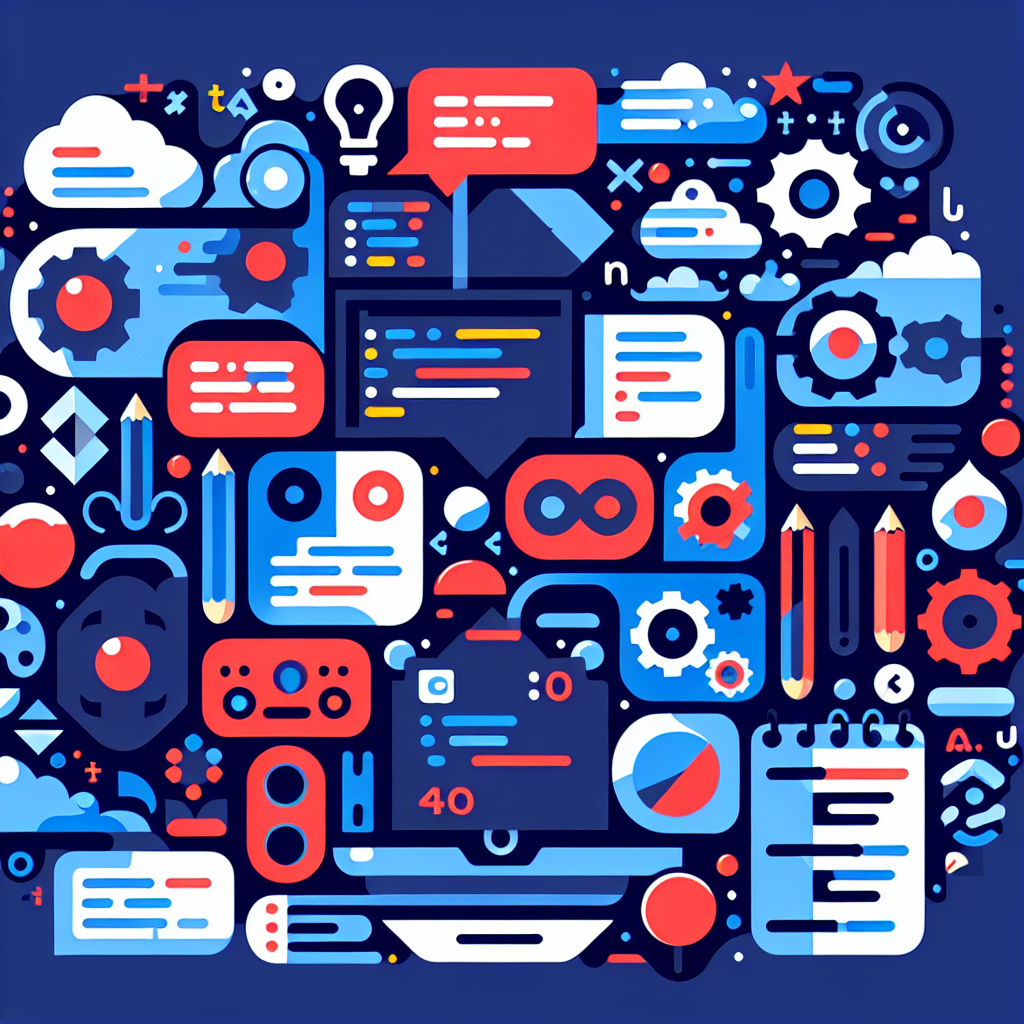
Implicit Binding: Effortless Elegance
Laravel performs a trick that feels as smooth as pulling a rabbit out of a hat – it automatically resolves Eloquent models for you. This sorcery, known as Implicit Binding, allows you to type-hint a model in your route or controller action, and it will be automatically resolved:
Route::get('/posts/{post}', function (App\Models\Post $post) {
return view('post.show', compact('post'));
});
Here, Laravel sees the 'post' parameter and marvelously fetches the corresponding 'Post' model. This not only reads like poetry but also brings forth a more secure and succinct way of handling data retrieval.
Customizing the Key, Mastering the Art
Implicit bindings typically use the model's primary key to find the model instance. However, true mastery comes from bending Laravel to your will by customizing route keys. You can direct Laravel to resolve a model based on another column such as a slug, resulting in cleaner and more readable URLs:
// In your Post model
public function getRouteKeyName() {
return 'slug';
}
Now, Laravel will recognize the 'slug' field as the parameter for resolving your binding. Abracadabra! The magic is complete, and your application has become more user-friendly.
Explicit Binding: Defining Your Destiny
For those seeking greater control, Laravel grants the power to declare explicit bindings. In this arcane process, you define exactly how the resolution should take place within the 'RouteServiceProvider':
public function boot()
{
parent::boot();
Route::model('custom_post', App\Models\Post::class);
}
In this spell, 'custom_post' explicitly tells Laravel which model to resolve, giving you the ability to customize the retrieval logic even further. This can be invaluable when your business logic demands specific behavior during model resolution.
Handling Missing Models with Grace
Not all heroes wear capes, and not all routes lead to a model. In cases where a model cannot be found for a given parameter, Laravel allows you to build a fallback strategy. Whether you want to show a 404 page or provide a default instance, Route Model Binding ensures your application's resilience:
Route::get('/posts/{post}', function (App\Models\Post $post = null) {
return $post ?? abort(404);
});
This way, you're prepared for the unexpected, and your application remains user-friendly and robust.
Route Model Binding in Resource Controllers
Route Model Binding and resource controllers are a duo that could only be matched by peanut butter and jelly. They are an impeccable match, as the binding also extends to controller methods, streamlining your CRUD operations within resource controllers:
public function show(App\Models\Post $post)
{
return view('posts.show', compact('post'));
}
This integration further empowers clarity and conciseness in your code, enhancing maintainability and readability.
Conclusion: Revel in the Craft of Route Model Binding
As you stand at the apex of Routing Fundamentals in Laravel, Route Model Binding emerges as a cornerstone of the efficient Laravel experience. By harnessing this elegant feature, you have now entered a realm of rapid and robust web application development. Revel in the newfound power and flexibility as you continue to weave the tapestry of your web dev mastery with Laravel.